FabricJS performance hack
The frame rate can be improved by only rendering inside a rAF callback.
The frame rate can be improved by only rendering inside a rAF callback.
let isRendering = false;
let isAnimating = false;
const render = canvas.renderAll.bind(canvas);
const stop = () => (isAnimating = false);
const play = () => {
isAnimating = true;
canvas.renderAll();
};
canvas.renderAll = () => {
if (!isRendering) {
isRendering = true;
requestAnimationFrame(() => {
render();
isRendering = false;
if (isAnimating) {
canvas.renderAll();
}
});
}
};
I started working with FabricJS this week so there's a big chance things might break. I couldn't find any place this might break after going through the canvas.renderAll method source code but this needs more tests before it can be used in any project. Here's screenshots of profiles before and after applying the hack on an example scene.
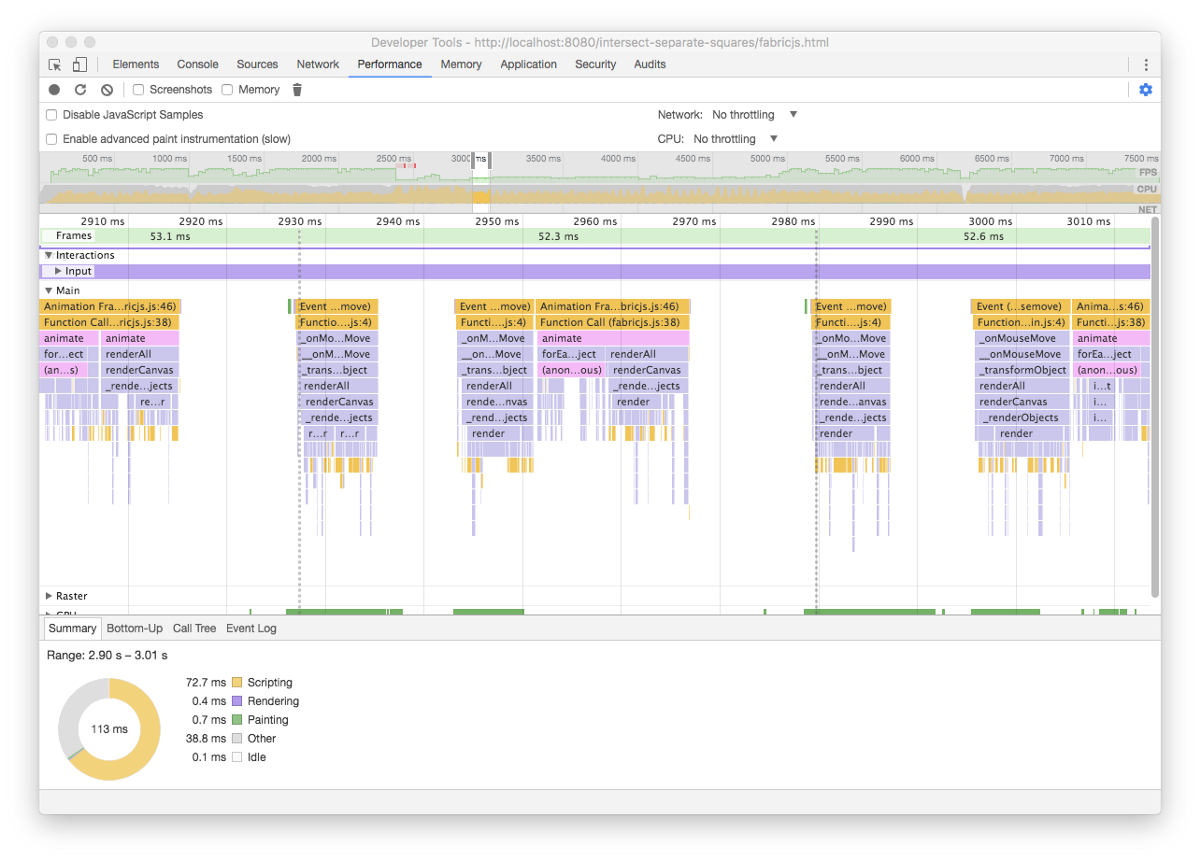
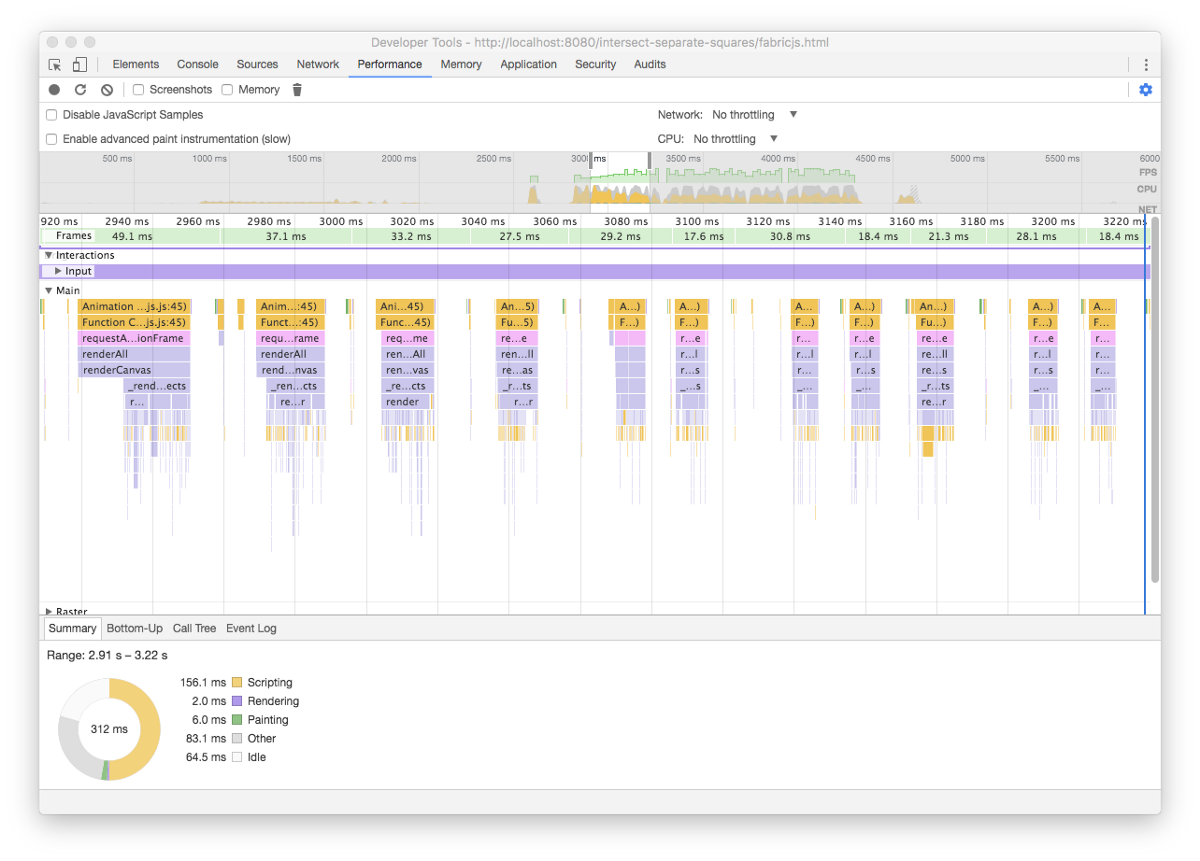
The frame rate can be improved further by running requestAnimationFrame in a loop ( example: using the play function in the gist ).
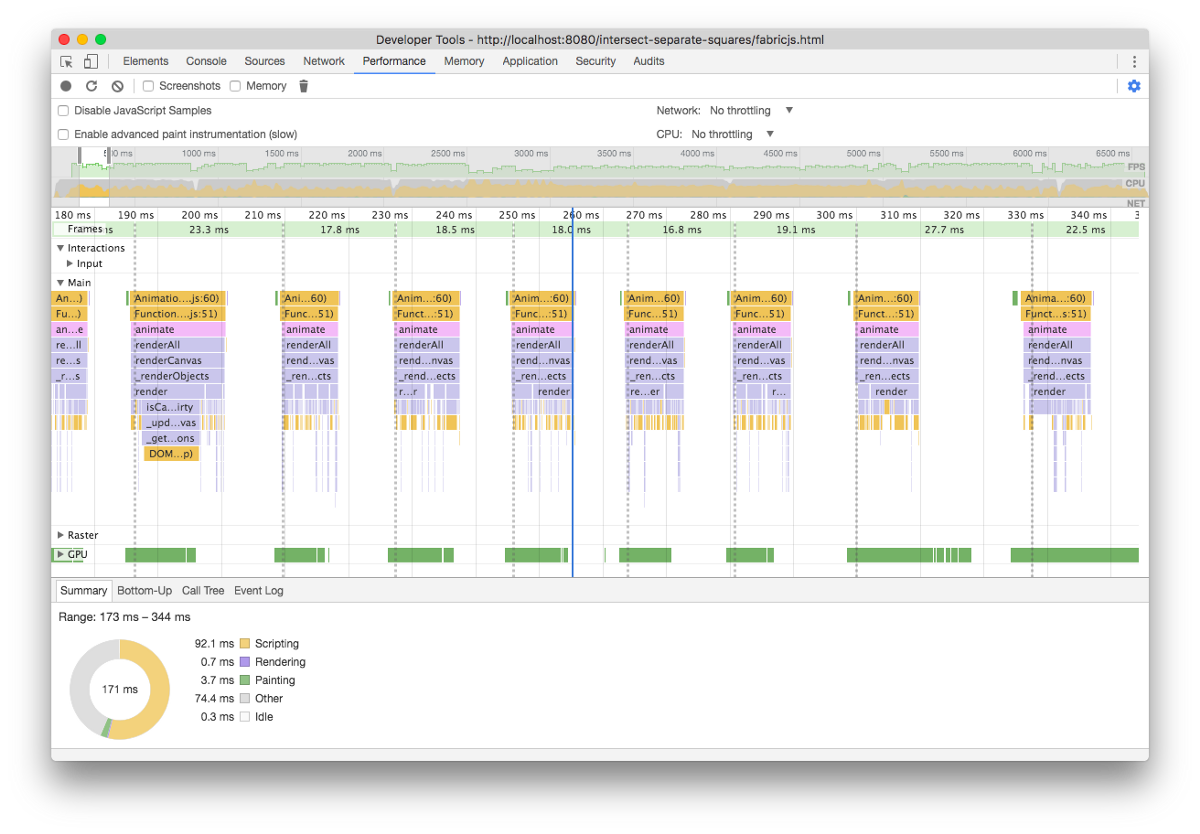
Even if this hack works, you'll need to do customize the hack depending on your application. Like I said, I've only started using FabricJS this week so if there's something wrong with the code, please let me know with a comment.